Introduction to Backend Frameworks
Backend frameworks are essential tools in the realm of web development, serving as the backbone for creating and managing the server-side of applications. These frameworks provide developers with a structured and efficient approach to handling the complexities associated with server-side logic, database interactions, and API (Application Programming Interface) development. By utilizing a backend framework, developers can focus on writing the core functionality of their applications rather than reinventing the wheel for every project.
One of the primary roles of backend frameworks is to facilitate the seamless interaction between a web application and its database. They offer various libraries and tools that simplify tasks such as data retrieval, storage, and updating, enabling developers to work with databases using simple and intuitive commands. Additionally, backend frameworks also support the creation of RESTful APIs, which are essential for enabling communication between the frontend and backend of web applications. These APIs allow for data exchange in real-time, enhancing user experience and overall application performance.
Furthermore, backend frameworks are crucial in maintaining security and managing user authentication. They often come with built-in features that help safeguard applications from common vulnerabilities, ensuring that sensitive data is protected. This aspect of backend frameworks is particularly vital in today’s digital landscape, where data breaches and security threats are increasingly prevalent.
While backend frameworks focus primarily on server-side operations, they are often contrasted with frontend frameworks, which handle the client-side aspects of applications. The distinction between these two types of frameworks is essential for developers, as it shapes the architecture of the entire web application. Understanding the role and significance of backend frameworks lays the groundwork for a comprehensive grasp of modern web development practices.
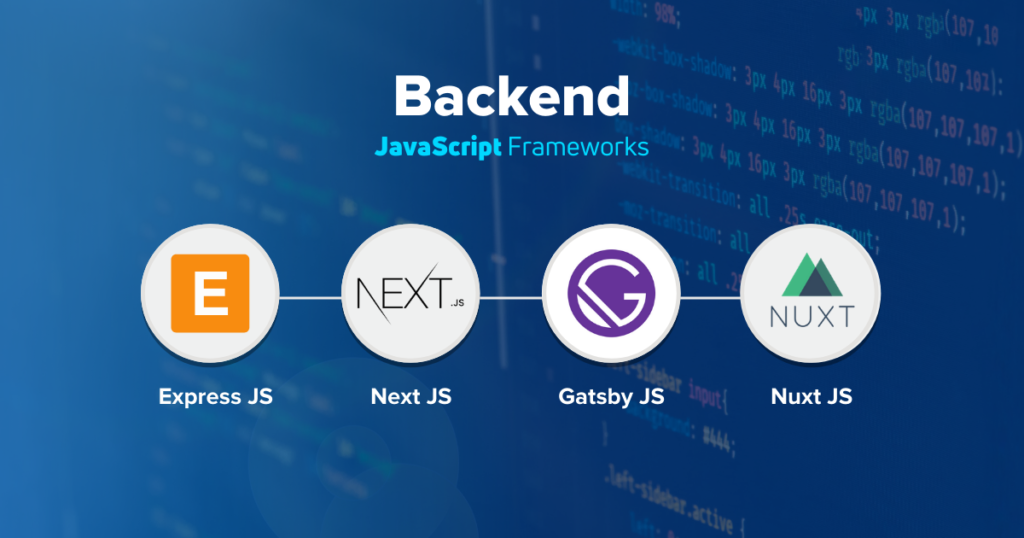
Types of Backend Frameworks
Backend frameworks can be categorized into three main types: monolithic frameworks, microservices frameworks, and serverless frameworks. Each type differs in structure, use cases, benefits, and limitations, thereby influencing the choice of framework based on specific project requirements.
Monolithic frameworks are characterized by a single unified codebase where all components are interconnected. This architecture is beneficial for smaller applications where rapid development and deployment are required. A monolithic structure allows for easier testing and debugging since the entire application is bundled. However, as applications scale, the monolithic approach can complicate updates and can lead to challenges in maintaining isolated components, making it less suitable for large systems.
Microservices frameworks, in contrast, adopt a distributed architecture, breaking down applications into individual services that can be developed, deployed, and scaled independently. This modular approach provides greater flexibility and makes it easier to manage complex applications, as each service can be updated without impacting the entire system. Microservices frameworks are appropriate for large-scale systems requiring responsiveness and continuous delivery. Nonetheless, they come with increased complexity in managing multiple services, including the need for effective inter-service communication and data consistency across the system.
Lastly, serverless frameworks represent an innovative approach to backend development, where developers do not need to manage server infrastructure. In this model, the cloud provider automatically scales and manages servers based on the needs of the application, which can lead to lower operational costs and reduced time to market. Serverless architectures are particularly suited for applications with unpredictable workloads. However, they may be restrictive if applications require complex processing or have long-running tasks, potentially leading to challenges in execution time limits and vendor lock-in.
Popular Backend Frameworks
Backend frameworks play a crucial role in web development by providing a structure for developing server-side applications. Some of the most popular backend frameworks currently in use include Node.js, Django, Ruby on Rails, and Flask, each offering unique features and capabilities to cater to various development needs.
Node.js is renowned for its non-blocking, event-driven architecture, facilitating scalable network applications. Built on JavaScript, it allows developers to use a single programming language for both frontend and backend development. This synergy enhances productivity and streamlines the development process. Notably, Node.js powers high-traffic applications, such as LinkedIn and Netflix, highlighting its capacity to handle large amounts of data efficiently.
Django, a Python-based framework, is celebrated for its “batteries-included” philosophy, providing a wide array of built-in features such as an ORM (Object-Relational Mapping) system and robust security measures. Its simplicity, combined with a well-structured admin interface, makes Django particularly appealing for developers looking to build powerful applications quickly. Major companies like Instagram and Spotify leverage Django’s capabilities to create dynamic web applications.
Ruby on Rails, often simply referred to as Rails, is a framework built on the Ruby programming language. It is designed to promote convention over configuration, which simplifies the development process. Rails is especially popular for startups and rapid application development due to its efficiency and strong community support. Notable projects like GitHub and Airbnb have been built using Ruby on Rails, underscoring its effectiveness in developing feature-rich applications.
Flask is a minimalistic Python-based framework that adopts a micro-framework approach. This design philosophy allows for flexibility and modularity, enabling developers to add components as needed. Flask is often used for smaller projects or as a basis for larger applications. Companies such as Pinterest and LinkedIn utilize Flask, demonstrating its versatility and ease of use.
Key Features to Look For in a Backend Framework
When selecting a backend framework, several critical features influence the decision-making process and overall effectiveness of development. Understanding these features not only aids in choosing a framework but also impacts long-term maintenance and scalability of applications.
One of the foremost considerations is performance. A backend framework should efficiently handle requests and process data without significant delays. This includes assessing the framework’s ability to manage traffic and execute queries quickly. High performance ensures a seamless user experience, which is vital for retention and satisfaction.
Another key feature is scalability. As applications grow, they should be capable of scaling to accommodate increased loads. A good backend framework offers tools and structures that allow developers to expand functionality effortlessly. This characteristic is essential for businesses aiming to adapt to changing demands and market conditions.
Security is also paramount. With increasing concerns over data breaches, a robust backend framework provides built-in security features such as authentication, authorization, and data protection measures. Assessing how a framework handles potential vulnerabilities can significantly influence an organization’s risk management strategy.
Developers also consider the ease of learning. A framework that is simple to understand allows for quicker onboarding of new team members and accelerates development timelines. A well-documented framework with clear tutorials and community resources can significantly reduce the learning curve and improve overall productivity.
Lastly, community support plays a vital role in choosing a backend framework. A strong community not only provides valuable resources and third-party integrations but also ensures ongoing updates and improvements. Active forums, comprehensive documentation, and user-generated content can assist developers in troubleshooting and enhancing their applications.
These features collectively shape the foundation upon which effective and secure applications are built, making them essential considerations for developers in a competitive landscape.
Setting Up a Backend Framework: A Step-by-Step Guide
Choosing a backend framework can significantly impact your development experience. In this guide, we will walk through the essential steps to set up a backend framework, using a widely used framework as an example.
The first step in setting up a backend framework involves installation. For this guide, we will use a popular framework such as Django for Python or Express for Node.js. In your terminal, you would begin by installing the necessary packages. For Django, run: pip install django
, and for Express, execute: npm install express
. This establishes the foundation for your application by providing you with the core functionality of the selected framework.
After installation, the next step is configuration. This process usually entails creating a new project. For Django users, you would run: django-admin startproject myproject
. For Express, you would create a directory and then initialize a Node.js application by using: npm init -y
. These commands set up the foundational structure of your project, complete with necessary configuration files.
A simple example project will illustrate the basic functionality provided by the backend framework. For example, if you are using Django, you can create an initial app within your project using: python manage.py startapp myapp
. This creates a directory structure tailored for your app where you may define models, views, and templates. Conversely, in an Express application, you can create a simple server by adding the following code snippet to the index.js
file:
const express = require('express');const app = express();const PORT = 3000;app.get('/', (req, res) => { res.send('Hello World!');});app.listen(PORT, () => { console.log(`Server is running on http://localhost:${PORT}`);});
Following these steps prepares your environment to build and test a simple backend application. Once the installation and initial configuration are complete, you can continue to develop your application by adding features, integrations, and exploring more advanced functionalities offered by the backend framework.
Backend Frameworks vs. Custom Development
When evaluating the choice between utilizing a backend framework and opting for custom development, several factors come into play that can significantly impact project timelines, resource allocation, and overall efficiency. Backend frameworks are pre-established tools that provide ready-made components and functionalities, allowing developers to create applications more rapidly. This can make frameworks an attractive option for projects with tight deadlines or limited budgets. By leveraging existing libraries and modules, developers can focus on customizing features rather than building them from scratch, which often results in a streamlined development process.
Frameworks come imbued with industry best practices, reducing the likelihood of security vulnerabilities and performance issues. For instance, popular frameworks such as Django or Ruby on Rails provide built-in security features, which can be advantageous for developers concerned about building secure applications. However, while frameworks may facilitate faster development, they may not always cater to specific business requirements. In cases where unique features or a high degree of customization is needed, custom development can prove to be the better option.
Custom development allows for complete control over every aspect of the application, enabling developers to implement unique functionalities that match specific business processes. It is especially beneficial for companies with specialized operations that standard frameworks may not support. However, custom development is typically more time-intensive and requires a higher investment in terms of resources and costs, resulting in potential delays in project delivery.
Ultimately, the decision to opt for a backend framework or custom development hinges on the project’s complexity, timeframe, and specific business needs. For straightforward applications that demand rapid deployment, frameworks may be ideal. In contrast, custom development is better suited for highly specialized applications where uniqueness is paramount.
Performance Optimization in Backend Frameworks
Performance optimization is a critical aspect of developing applications using backend frameworks, as it directly impacts user experience and system efficiency. One key technique for enhancing performance is caching. Caching involves storing frequently accessed data in a temporary storage area, which reduces the need for repetitive database calls. Implementing caching strategies, such as in-memory caches and distributed caching systems, can dramatically decrease response times and improve overall application responsiveness.
Database optimization is another fundamental technique that can lead to significant performance improvements. This includes using proper indexing, selecting only the necessary data, and structuring queries efficiently. By examining query execution plans, developers can identify bottlenecks and make adjustments that will enhance data retrieval speeds. Additionally, employing techniques such as database normalization and denormalization can help streamline data storage and access, depending on the application’s requirements.
Code splitting is also a crucial aspect of performance optimization in backend frameworks. This process involves breaking down code into smaller, manageable pieces, which allows for efficient loading and execution. By utilizing modern build tools, developers can implement dynamic imports that ensure that only the necessary code is loaded at any given time, reducing the initial load time and improving user interaction.
Asynchronous programming has become increasingly popular for optimizing backend performance. This approach allows multiple tasks to run concurrently, which can significantly improve application throughput. Leveraging asynchronous functions in the backend can minimize blocking and maximize resource utilization, resulting in more responsive applications. However, it is essential to be mindful of potential pitfalls, such as callback hell and error handling complexities, which can complicate code maintenance.
In conclusion, employing these performance optimization techniques—caching strategies, database optimization, code splitting, and asynchronous programming—can lead to significant enhancements in applications built on backend frameworks. By adhering to best practices and avoiding common pitfalls, developers can ensure that their applications function efficiently and effectively.
Trends and Future of Backend Frameworks
The landscape of backend frameworks is continually evolving, influenced by emerging technologies and changing developer needs. One of the most notable trends in recent years is the rise of JAMstack architecture, which emphasizes client-side operations, reusable APIs, and pre-rendered markup. This approach allows developers to build fast and secure applications by decoupling the frontend from the backend. By leveraging static site generators and headless CMS, the JAMstack paradigm provides numerous advantages, including improved performance and enhanced scalability.
Along with JAMstack, GraphQL has gained traction as a powerful alternative to traditional REST APIs. This query language enables clients to specify the structure of the data they require, reducing over-fetching and making APIs more efficient and flexible. Consequently, GraphQL facilitates faster development cycles and streamlined communication between frontend and backend integrations, becoming a preferred choice for many modern applications.
Another significant movement is towards cloud-native architecture, which emphasizes the use of cloud computing to build and manage applications. With the increasing adoption of microservices, organizations can deploy and scale their backend components independently. This modular design enhances resource utilization, fosters greater resilience, and allows for faster implementation of new features. As businesses begin to embrace the capabilities of cloud providers, the demand for cloud-native tools and frameworks is expected to rise.
Looking to the future, the emphasis on automation and developer experience is likely to reshape backend development. Technologies such as serverless computing will increasingly become mainstream, allowing developers to focus on writing code without worrying about infrastructure management. As artificial intelligence and machine learning become more prevalent, they may also influence the way backend frameworks are designed, optimizing performance and data handling in real-time. Overall, the trends within backend frameworks indicate a shift towards greater efficiency, scalability, and flexibility, ensuring that they remain responsive to the demands of modern software development.
Resources for Learning Backend Frameworks
As the demand for proficient developers continues to grow, various resources are available to facilitate the mastery of backend frameworks. These resources cater to different learning preferences and skill levels, ensuring that both beginners and experienced developers can effectively enhance their knowledge and skills in backend development.
For novice developers, beginner-friendly books such as “Learning PHP, MySQL & JavaScript” by Robin Nixon offer a comprehensive introduction to essential backend concepts. Furthermore, “Flask Web Development” by Miguel Grinberg provides a practical guide to creating applications using the Flask framework. These texts not only cover foundational principles but also provide hands-on projects that reinforce learning.
Online courses are another valuable resource. Platforms like Coursera and Udemy feature comprehensive courses tailored specifically for backend frameworks like Django, Ruby on Rails, and Node.js. For instance, the “Node.js – The Complete Guide” course on Udemy thoroughly introduces developers to server-side JavaScript programming, enabling learners to build robust applications.
Tutorial websites such as freeCodeCamp and W3Schools serve as excellent starting points, as they offer structured lessons and real-world projects. Additionally, GitHub and Stack Overflow have vibrant community forums where developers can seek help, share knowledge, and collaborate on projects. These platforms often feature repositories containing sample codes and frameworks that can further aid in the learning process.
For those who prefer interactive learning, websites such as Codecademy provide coding exercises and projects specifically for backend frameworks, allowing users to practice in real time. This hands-on approach can significantly bolster understanding and retention of complex concepts.
In conclusion, a variety of resources are available to aid in the learning of backend frameworks, ranging from books and online courses to community forums and interactive websites. By utilizing these tools, developers can effectively deepen their knowledge and successfully enhance their skills in backend development. Each resource plays a vital role in building a solid foundation for a successful career in this field.