Introduction to Backend Development
Backend development is a crucial component of web development that focuses on server-side functionalities, ensuring smooth and efficient operation of websites and applications. While frontend development handles the visual aspects of a website—what users see and interact with—the backend manages the data exchange, server configuration, and application logic that function in the background. This division of responsibilities is essential for building dynamic, user-friendly interfaces while maintaining robust performance.
At its core, backend development involves several key elements: servers, databases, and application programming interfaces (APIs). Servers act as the backbone of a web application, receiving requests from the client-side, processing them, and returning the appropriate responses. These servers are powered by programming languages such as Python, Ruby, Java, and PHP, each offering unique features to tackle specific backend challenges.
Databases are another critical aspect of backend development. They serve as storage systems that manage and organize data, allowing for efficient retrieval and manipulation. Common database technologies include relational databases like MySQL and PostgreSQL, and NoSQL databases such as MongoDB. Understanding these databases is vital as they directly affect data accessibility and performance.
APIs, on the other hand, enable communication between different software systems, allowing them to exchange data and functionality seamlessly. Through RESTful services or GraphQL, APIs provide a layer that facilitates interactions between the frontend and backend, ensuring that users receive the most updated information on demand.
In summary, backend development plays an indispensable role in the creation of any web application. Its focus on server-side logic, data organization, and integration through APIs ensures that users enjoy a seamless experience while interacting with web applications. The interplay of these core components lays the foundation for understanding more complex backend development practices and technologies.
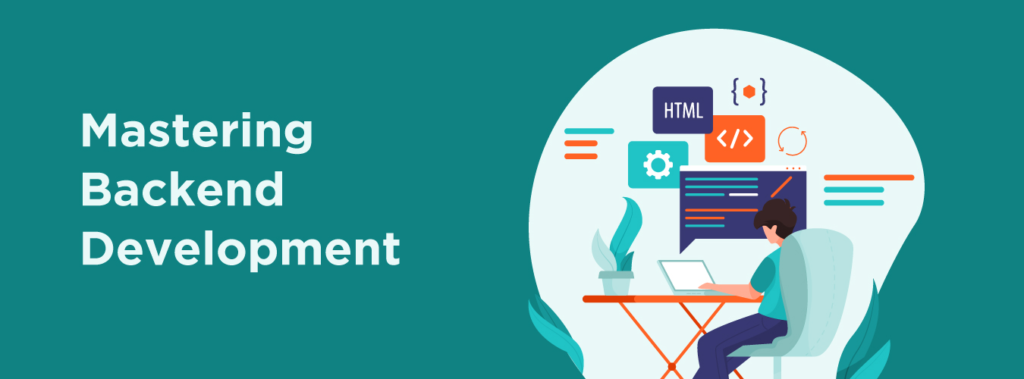
Key Technologies in Backend Development
Backend development plays a crucial role in powering applications and websites, with various programming languages and frameworks offering distinct advantages. In this segment, we will examine some of the most prevalent backend technologies, emphasizing their strengths, weaknesses, use cases, and community backing. Understanding these elements can guide developers in selecting the right technology for their projects.
Node.js has emerged as a popular choice for backend development due to its non-blocking, event-driven architecture. This JavaScript runtime environment enables developers to build scalable network applications efficiently. One significant advantage of Node.js is its ability to handle numerous connections simultaneously, making it ideal for real-time applications such as chat platforms. However, its single-threaded nature may present challenges when handling heavy computation tasks.
Python is another widely-used programming language in backend development, primarily utilized through frameworks such as Django and Flask. Django, with its “batteries-included” philosophy, provides extensive features out-of-the-box, allowing developers to quickly build high-quality web applications. On the other hand, Flask offers a micro-framework approach, granting developers greater flexibility and control over their applications. While Python’s readability and ease of learning are significant advantages, it may not perform as well as some compiled languages in high-load scenarios.
Ruby on Rails is a robust framework that emphasizes convention over configuration, allowing developers to focus on building applications rather than dealing with complex setup processes. It promotes rapid development through its extensive library of reusable components. However, Ruby’s performance can sometimes lag behind other technologies, particularly when scaling applications to handle increased traffic.
Lastly, PHP remains a staple in backend development, especially for content management systems like WordPress. Although often criticized for inconsistent syntax and performance issues, PHP’s vast ecosystem of frameworks, such as Laravel and Symfony, has improved its status. PHP’s ease of deployment and strong community support make it a viable option for many developers. Ultimately, the choice of technology will largely depend on the specific requirements of each project, including scalability, speed, and team expertise.
Frameworks and Libraries
Backend development has significantly evolved with the emergence of various frameworks and libraries that facilitate and streamline the coding process. These tools offer developers predefined functionalities and protocols, thus enabling them to focus on building robust applications efficiently. Among the most popular frameworks, Express.js stands out as a prominent choice for Node.js. It is characterized by its minimalistic and unopinionated design, allowing developers to implement routing and middleware easily. This flexibility makes Express.js ideal for building scalable applications and APIs, particularly for real-time data exchange scenarios.
In the realm of Python, Django serves as a powerful web framework tailored for rapid development. Its “batteries-included” architecture provides built-in features like an admin panel, ORM (Object Relational Mapping), and robust security, which significantly reduce development time and enhance the security of applications. Django’s emphasis on reusability promotes the use of components that can be easily plugged into new projects, thus ensuring developers adhere to the DRY (Don’t Repeat Yourself) principle.
For PHP developers, Laravel has emerged as a leading framework due to its expressive syntax and extensive suite of tools. One of its standout features is Eloquent ORM, which simplifies database management through an intuitive API, thereby reducing the amount of boilerplate code required. Additionally, Laravel embraces modern development practices, offering built-in features like task scheduling, event broadcasting, and unit testing, which improve overall productivity.
Beyond frameworks, libraries play a crucial role in backend development by providing reusable components that save time and ensure quality. Libraries such as Axios for handling HTTP requests, or Lodash for utility functions, enhance the development workflow by allowing developers to integrate pre-built solutions into their projects. Ultimately, leveraging frameworks and libraries not only speeds up the development process but also ensures adherence to best practices in coding.
Databases and Data Management
In the realm of backend development, databases play a pivotal role in managing and organizing data efficiently. They are essential for storing, retrieving, and manipulating information, ultimately serving as the backbone of most applications. Two primary categories of databases dominate the field: SQL and NoSQL. Understanding the differences between these database types is crucial for any developer.
SQL databases, such as MySQL and PostgreSQL, are structured and utilize a predefined schema to define data types and relationships. Their advantages include strong consistency, a robust querying language (SQL), and support for complex transactions. This makes them highly suitable for applications that require precise data integrity and where relationships between data sets are intricate. However, they can have drawbacks, including scalability issues in handling large volumes of unstructured data and a rigidity that may hinder rapid development.
On the other hand, NoSQL databases like MongoDB and Cassandra offer a flexible schema design, allowing for various data structures such as documents, key-value pairs, and wide-column stores. These databases excel in horizontal scaling and are adept at managing large volumes of unstructured or semi-structured data, which is increasingly common in modern applications. However, NoSQL databases may sacrifice some level of consistency, and the lack of a standardized querying language can complicate data retrieval depending on the specific NoSQL offering.
In addition to choosing between SQL and NoSQL databases, backend developers often utilize Object-Relational Mappers (ORMs) to facilitate data interactions. ORMs are tools that streamline the process of working with databases by allowing developers to interact with database entities as objects within their programming language, thus enhancing productivity and reducing the likelihood of errors. They abstract the complexities of database commands and offer a more intuitive approach to building data-driven applications.
APIs and Their Importance
Application Programming Interfaces, commonly referred to as APIs, serve as crucial components in backend development by enabling communication between different software applications. They define a set of rules and protocols that allow various systems to interact, ensuring data exchange and functionality across diverse platforms. The significance of APIs in modern web development cannot be overstated, as they facilitate integration, enhance system interoperability, and enable developers to leverage existing functionality rather than building everything from scratch.
One of the most prevalent architectures used for APIs today is Representational State Transfer (REST). RESTful APIs utilize standard HTTP methods—such as GET, POST, PUT, and DELETE—to perform operations on resources identified by URLs. They are lightweight, simple to use, and widely adopted due to their stateless nature, making them an ideal choice for web services. Alternatively, GraphQL has emerged as a powerful query language for APIs, providing clients with the flexibility to request only the data they need. This can significantly reduce over-fetching and under-fetching of data, contributing to improved performance and efficient data management.
Creating and consuming APIs effectively involves several best practices. Developers should focus on proper versioning, ensuring clients remain compatible as changes are made. Consistent and clear documentation is essential, as it enables users to understand how to interact with the API and implement it effectively. Moreover, security must be a top priority. Employing methods such as token-based authentication, adopting HTTPS, and validating input can safeguard APIs against common threats.
In conclusion, APIs are foundational to backend development, enabling seamless integration and communication between applications. By understanding their significance and adhering to best practices for creation, consumption, and security, developers can enhance their ability to build robust and scalable systems that meet the demands of modern digital environments.
Building a Scalable Backend
Scalability is a crucial aspect of backend development, particularly for applications that anticipate increasing loads and user traffic over time. A well-thought-out backend architecture enables services to adapt seamlessly to varying demands without compromising performance or reliability. There are several strategies to achieve a scalable backend, each serving a specific purpose in managing system capacity.
One fundamental technique is load balancing, which distributes incoming traffic across multiple servers. This distribution prevents any single server from becoming a bottleneck, thereby enhancing both response times and overall system reliability. Load balancers can also monitor the health of servers, automatically redirecting traffic away from those that may be down or overloaded. By utilizing load balancing, backend systems can dynamically manage increased traffic while maintaining responsiveness.
Another vital strategy is the implementation of caching mechanisms. Caching allows frequently accessed data to be stored temporarily in a fast-access layer, reducing the time required for database queries. Leveraging technologies such as Redis or Memcached can significantly improve the performance of your backend by minimizing latency and lowering database load. This is particularly effective for read-heavy applications where data doesn’t frequently change.
Adopting a microservices architecture is also instrumental in building a scalable backend. This architectural style breaks down applications into smaller, independent services that can be developed, deployed, and scaled individually. This not only simplifies the development process but also allows for easier management of each service, making it possible to scale specific components based on their load requirements without affecting the entire system.
Lastly, containerization using tools like Docker and orchestration through Kubernetes further enhances the scalability of applications. Containers encapsulate application components and their dependencies, ensuring consistency across various environments. Kubernetes assists in automating deployment, scaling, and management of containerized applications, enabling seamless scaling in response to demand fluctuations. By leveraging these modern technologies, developers can build robust and scalable backends capable of handling increased loads efficiently.
Security Practices in Backend Development
Ensuring robust security in backend development is critical for protecting sensitive data and maintaining the trust of users. A multi-layered approach to security can help safeguard applications from potential threats and vulnerabilities. One of the foundational practices in backend security is the implementation of strict network security measures. This includes utilizing firewalls, virtual private networks (VPNs), and intrusion detection systems to monitor and control incoming and outgoing network traffic. By establishing these controls, developers can mitigate the risk of unauthorized access and data breaches.
Another key aspect of backend security involves the use of reliable authentication and authorization mechanisms. Implementing protocols such as OAuth and JSON Web Tokens (JWT) can significantly enhance security. OAuth, for example, allows users to authorize third-party applications to access their data without sharing their passwords, while JWT provides a secure way to transmit information between parties as a JSON object. Properly managing user sessions and access tokens is vital to prevent session hijacking and unauthorized user access.
Data encryption is crucial for safeguarding sensitive information both at rest and in transit. Utilizing encryption algorithms ensures that even if data is intercepted, it remains unreadable to unauthorized users. Techniques such as Transport Layer Security (TLS) are essential for encrypting data during transmission between clients and servers, while encryption at rest secures stored data from breaches. Additionally, backend developers must guard against common vulnerabilities, including SQL injection and cross-site scripting (XSS). By employing input validation, using prepared statements, and escaping output, developers can significantly reduce the surface area for potential attacks.
In conclusion, integrating these essential security practices into backend development will help create a resilient application. Effective strategies, including network security protocols, robust authentication mechanisms, data encryption, and protection against vulnerabilities, are imperative for building secure backend systems. Adhering to these practices ensures that applications can withstand various threats while maintaining data integrity and user trust.
Testing and Debugging Your Backend System
Testing and debugging are critical components of backend development, as they ensure functionalities work as intended, thereby leading to a robust system. Effective testing involves various methodologies, primarily unit tests, integration tests, and end-to-end tests. Each of these testing types serves a unique purpose within the development process. Unit tests are aimed at validating individual components, ensuring each function correctly performs its designated task. By conducting unit tests early in the development, developers can detect issues before they escalate, which enhances the overall quality of the backend.
Integration tests, on the other hand, examine the connections between various system components, verifying that they collaborate seamlessly. This type of testing is vital, especially when multiple services interact within a microservices architecture. Lastly, end-to-end tests simulate real user scenarios, confirming that all components, from the user interface to the database, function together as expected. Implementing a combination of these testing strategies aids in identifying problems across different layers of the backend system.
To efficiently conduct these tests, various tools come into play. For instance, Postman is widely recognized for API testing, allowing developers to ensure that endpoints respond correctly under various conditions. Jest, a JavaScript testing framework, simplifies the process of writing and running unit tests, making it popular among developers of Node.js applications. Other tools such as Mocha and Cypress provide additional functionalities for enhanced testing experiences.
Debugging techniques further augment the chance of achieving a bug-free backend. Employing logging practices allows developers to track the flow of a program and identify areas where errors occur. Utilizing tools like Node.js’ built-in debugger helps isolate and address issues in real-time. The combination of thorough testing and effective debugging cultivates confidence in the backend, ensuring seamless operations that enhance user satisfaction.
Future Trends in Backend Development
As the technological landscape continues to evolve, several emerging trends in backend development are shaping its future. One notable trend is the rise of serverless architecture, which allows developers to build and run applications without the need to manage server infrastructure. This paradigm shift enables teams to focus more on coding and less on server management, improving scalability and reducing costs. Serverless computing also facilitates faster deployment, further accelerating development cycles. With cloud providers offering integrated solutions, the adoption of serverless architecture is poised to grow significantly.
Another key trend gaining traction is the increasing popularity of GraphQL, a query language for APIs that allows clients to request only the data they need. This flexibility contrasts with traditional RESTful APIs, which often return fixed data structures. As applications become more complex, developers are turning to GraphQL for its efficiency and ease of use. The type system and introspective capabilities of GraphQL enhance the developer experience, making it easier to define and validate queries. As a result, we can expect to see wider adoption of GraphQL as a core technology for backend systems.
Additionally, the integration of artificial intelligence (AI) and machine learning (ML) into backend systems is transforming the way applications operate. By leveraging AI and ML technologies, developers can optimize their applications for performance, enhance user experiences, and automate repetitive tasks. For instance, AI-driven algorithms can analyze user data to provide personalized recommendations, while ML models can improve data processing capabilities. As businesses increasingly seek to harness the power of data, the use of AI and ML in backend development will likely become a standard practice.
In summary, the future of backend development is poised for significant changes, driven by trends such as serverless architecture, GraphQL, and AI and ML integration. By staying abreast of these developments, developers can ensure they are equipped to meet the evolving demands of the digital landscape.